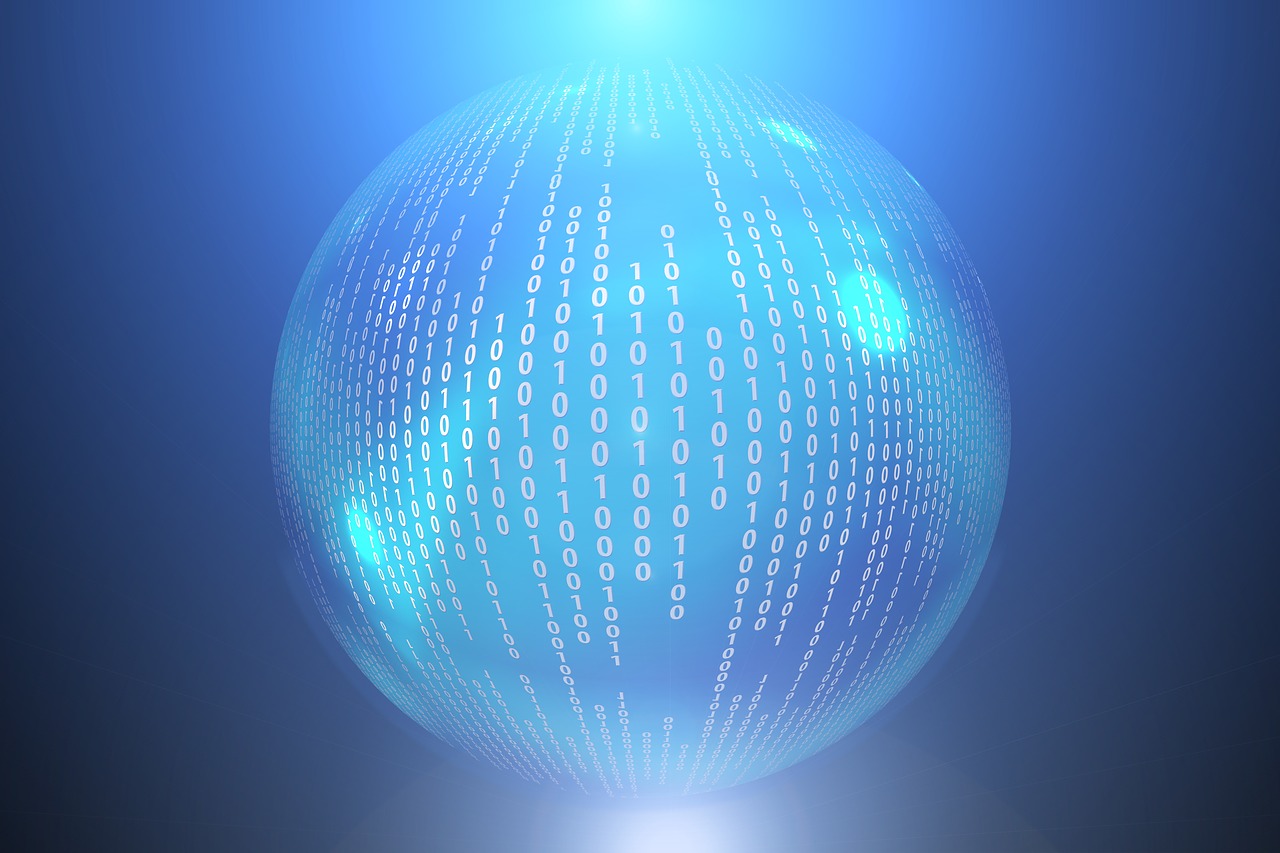
The outline and initial setup method of Python are as follows. These will prepare you for Python.
Then, We describe how to run a python app as a starting point for Python programming. In addition, we also describe string output, calculations, and comment.
See below for other Python knowledge.
Sponsored Links
Create and run Python source file
The Python source file extension is “.py” .
Linux
- Describe the following printf() function in Emacs or vi, and save it with an arbitrary file name (e.g., poc0001.py).
print("Hello World!")
- Execute the following command on the saved file on the command line.
- Confirm that the entered character string is output as shown below.
$ python3 poc0001.py
Hello World!
Windows
- Describe the following printf() function in Notepad or Wordpad, and save it with an arbitrary file name (e.g., poc0001.py).
print("Hello World!")
- Execute the following command on the saved file on the command prompt or PowerShell.
- Confirm that the entered character string is output as shown below.
$ python poc0001.py
Hello World!
String output and Calculation
- Create a source file that describes the following (poc0002.py).
print("4*2 = " + str(4 * 2))
- Execute with the following command.
- For Windows, replace “python3” with “python” and execute.
$ python3 poc0002.py
4*2 = 8
- We explain the result of the above statement.
- First, 4 * 2 in the str() function is treated as a number and a multiplication symbol, so it is calculated and becomes the number 8.
- Since the str() function is a function that replaces a numerical value with a character string, 8 as the above numerical value is replaced with “8” as a character string.
- The area enclosed in ” ” in the print() function is treated as a character string. Furthermore, + in the print() function concatenates the strings before and after it.
- From the above results, “4 * 2 =” is output as it is, and str (4 * 2) is output as the character “8”. As a result, the above output is obtained.
String output control
Character string output without line breaks
- Modify the source file (poc0002.py) and make it as follows.
print("4*2 = " + str(4 * 2))
print("!" + "?")
- Execute with the following command.
- For Windows, replace “python3” with “python” and execute.
$ python3 poc0002.py
4*2 = 8
!?
- As the execution result, the result of the statement on the first line includes a line break, so the result of the statement on the second line that follows is output on the second line.
- Modify the source file (poc0002.py) and make it as follows.
print("4*2 = " + str(4 * 2), end="")
print("!" + "?")
- Execute with the following command.
- For Windows, replace “python3” with “python” and execute.
$ python3 poc0002.py
4*2 = 8!?
- By using end = “” as an argument of the print() function, such as print (argument 1, end = “”), it is possible to output without line breaks.
Character string output with line breaks
- Create a source file that describes the following (poc0003.py) .
print("""2+4
=
6""" + "!!!")
- Execute with the following command.
- For Windows, replace “python3” with “python” and execute.
$ python3 poc0003.py
2+4
=
6!!!
- If you enclose the character string part in triple double quotation marks as described above, the character string output will include line breaks.
Add comment
- Comments are indispensable for improving readability in source code development.
- One-line comments in Python are represented by #.
- There is no such thing as a multi-line comment in Python. However, in Python, if you write a character string, numerical value, or calculation formula without using a function, an error will not occur and it will be ignored. Taking advantage of its characteristics, you can use it like a multi-line comment by writing a character string with triple double quotation marks.
- Create a source file that describes the following (poc0004.py) .
# comment
print("test") # test2
print("!" + "?")
""" This
is
a test comment.
"""
"test3"
3
1+2
- Execute with the following command.
- For Windows, replace “python3” with “python” and execute.
$ python3 poc0004.py
test
!?
- As mentioned above, the string from # to the end of the line is treated as a comment.
- As mentioned above, the string including line breaks enclosed in triple double quotes is ignored and treated like a comment.
- As mentioned above, the character string “test3”, the numerical value 3, and the formula 1 + 2 that are not used in the function are all ignored.
Sponsored Links